Python Read Numbers Text File Add Sum
Overview
When yous're working with Python, you don't need to import a library in order to read and write to a file. Information technology's handled natively in the linguistic communication, albeit in a unique manner. Below, we outline the simple steps to read and write to a file in Python.
The offset thing you lot'll need to exercise is use the built-in python open up file function to get a file object .
The open role opens a file. It's uncomplicated. This is the get-go footstep in reading and writing files in python.
When yous use the open function, it returns something called a file object . File objects contain methods and attributes that can be used to collect information about the file you opened. They can also be used to manipulate said file.
For case, the mode attribute of a file object tells you which mode a file was opened in. And the proper noun attribute tells you lot the name of the file.
Yous must understand that a file and file object are two wholly separate – yet related – things.
File Types
What you may know every bit a file is slightly different in Python.
In Windows, for example, a file can be any item manipulated, edited or created by the user/Bone. That means files can be images, text documents, executables, and excel file and much more than. Most files are organized by keeping them in individual folders.
A file In Python is categorized as either text or binary, and the difference between the 2 file types is of import.
Text files are structured as a sequence of lines, where each line includes a sequence of characters. This is what you lot know equally lawmaking or syntax.
Each line is terminated with a special graphic symbol, called the EOL or Cease of Line character. There are several types, but the almost common is the comma {,} or newline character. It ends the electric current line and tells the interpreter a new one has begun.
A backslash character tin can likewise exist used, and information technology tells the interpreter that the side by side character – following the slash – should be treated as a new line. This character is useful when you lot don't want to start a new line in the text itself but in the code.
A binary file is whatever blazon of file that is non a text file. Because of their nature, binary files tin only be processed by an application that know or understand the file'south structure. In other words, they must be applications that tin can read and interpret binary.
Reading Files in Python
In Python, files are read using the open() method. This is ane of Python's congenital-in methods, fabricated for opening files.
The open() function takes two arguments: a filename and a file opening mode. The filename points to the path of the file on your computer, while the file opening mode is used to tell the open() function how nosotros plan to interact with the file.
By default, the file opening mode is set to read-just, meaning we'll only have permission to open and examine the contents of the file.
On my figurer is a folder called PythonForBeginners. In that folder are three files. One is a text file named emily_dickinson.txt, and the other two are python files: read.py and write.py.
The text file contains the following poem, written by poet Emily Dickinson. Possibly we are working on a poetry program and have our poems stored as files on the computer.
Success is counted sweetest
By those who ne'er succeed.
To cover a nectar
Requires sorest need.
Not i of all the Purple Host
Who took the Flag today
Can tell the definition
And then articulate of Victory
As he defeated, dying
On whose forbidden ear
The distant strains of triumph
Outburst agonized and clear.
Before nosotros can do anything with the contents of the poem file, we'll need to tell Python to open it. The file read.py, contains all the python code necessary to read the poem.
Any text editor tin can be used to write the code. I'yard using the Atom code editor, which is my editor of choice for working in python.
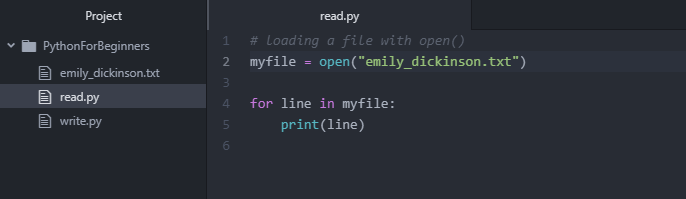
# read.py # loading a file with open() myfile = open("emily_dickinson.txt") # reading each line of the file and printing to the console for line in myfile: print(line)
I've used Python comments to explicate each pace in the code. Follow this link to acquire more about what a Python comment is.
The case above illustrates how using a elementary loop in Python can read the contents of a file.
When it comes to reading files, Python takes care of the heaving lifting behind the scenes. Run the script by navigating to the file using the Command Prompt — or Concluding — and typing 'python' followed past the name of the file.
Windows Users: Before y'all can use the python keyword in your Command Prompt, you'll need to set up the environment variables. This should have happened automatically when you installed Python, just in example it didn't, you may need to do information technology manually.
>python read.py
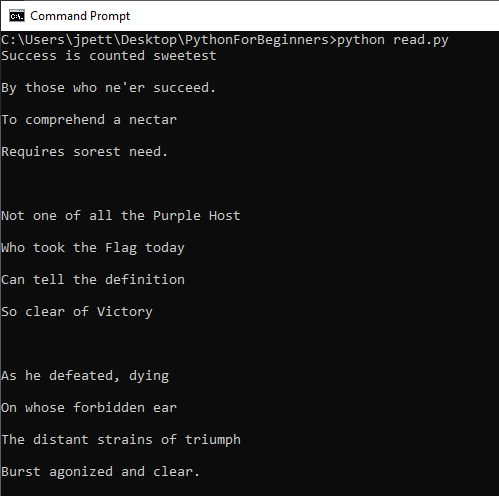
Data provided past the open() method is unremarkably stored in a new variable. In this case, the contents of the verse form are stored in the variable "myfile."
In one case the file is created, nosotros can use a for loop to read every line in the file and print its contents to the command line.
This is a very simple example of how to open a file in Python, merely student's should be aware that the open up() method is quite powerful. For some projects it will be the but thing needed to read and write files with Python.
Writing Files in Python
Before nosotros can write to a file in Python, it must first exist opened in a unlike file opening way. We can do this by supplying the open() method with a special argument.
In Python, write to file using the open up() method. You'll need to pass both a filename and a special character that tells Python we intend to write to the file.
Add the following code to write.py. We'll tell Python to look for a file named "sample.txt" and overwrite its contents with a new message.
# open the file in write manner myfile = open("sample.txt",'w') myfile.write("How-do-you-do from Python!")
Passing 'w' to the open() method tells Python to open up the file in write way. In this style, any information already in the file is lost when the new data is written.
If the file doesn't exist, Python will create a new file. In this case, a new file named "sample.txt" volition be created when the program runs.
Run the program using the Command Prompt:
>python write.py
Python tin also write multiple lines to a file. The easiest way to do this is with the writelines() method.
# open the file in write mode myfile = open("sample.txt",'west') myfile.writelines("Hi World!","Nosotros're learning Python!") # close the file myfile.shut()
We can likewise write multiple lines to a file using special characters:
# open the file in write way myfile = open up("verse form.txt", 'west') line1 = "Roses are crimson.\due north" line2 = "Violets are blueish.\n" line3 = "Python is slap-up.\due north" line4 = "And and so are you.\n" myfile.write(line1 + line2 + line3 + line4)
Using string concatenation makes information technology possible for Python to save text data in a diversity of ways.
However, if nosotros wanted to avoid overwriting the data in a file, and instead suspend it or change information technology, we'd have to open up the file using some other file opening fashion.
File Opening Modes
By default, Python will open the file in read-only mode. If we want to do anything other than just read a file, nosotros'll demand to manually tell Python what we intend to do with it.
- 'r' – Read Mode: This is the default mode for open() . The file is opened and a pointer is positioned at the beginning of the file's content.
- 'west' – Write Mode: Using this mode volition overwrite any existing content in a file. If the given file does not exist, a new one will be created.
- 'r+' – Read/Write Mode: Use this way if you demand to simultaneously read and write to a file.
- 'a' – Append Mode: With this mode the user can suspend the information without overwriting any already existing data in the file.
- 'a+' – Append and Read Fashion: In this fashion you can read and suspend the data without overwriting the original file.
- '10' – Exclusive Creating Mode: This mode is for the sole purpose of creating new files. Employ this mode if yous know the file to be written doesn't exist beforehand.
Notation: These examples assume the user is working with text file types. If the intention is to read or write to a binary file blazon, an additional argument must be passed to the open() method: the 'b' character.
# binary files need a special argument: 'b' binary_file = open("song_data.mp3",'rb') song_data = binary_file.read() # close the file binary_file.close()
Closing Files with Python
Subsequently opening a file in Python, it's important to close it afterwards you're washed with it. Closing a file ensures that the program can no longer access its contents.
Close a file with the close() method.
# open a file myfile = open("poem.txt") # an assortment to store the contents of the file lines = [] For line in myfile: lines.suspend(line) # shut the file myfile.close() For line in liens: print(line)
Opening Other File Types
The open up() method can read and write many dissimilar file types. We've seen how to open up binary files and text files. Python can too open images, allowing you lot to view and edit their pixel data.
Before Python can open an prototype file, the Pillow library (Python Imaging Library) must exist installed. It's easiest to install this module using pip.
pip install Pillow
With Pillow installed, Python can open prototype files and read their contents.
From PIL import Image # tell Pillow to open up the paradigm file img = Paradigm.open("your_image_file.jpg") img.show() img.shut()
The Pillow library includes powerful tools for editing images. This has made it one of the well-nigh popular Python libraries.
With Statement
Yous can also piece of work with file objects using the with statement. It is designed to provide much cleaner syntax and exceptions treatment when you are working with code. That explains why it's good practice to employ the with statement where applicable.
I bonus of using this method is that any files opened will be closed automatically after y'all are done. This leaves less to worry about during cleanup.
To utilize the with statement to open a file:
with open("filename") as file:
Now that y'all understand how to call this statement, let's take a wait at a few examples.
with open("poem.txt") every bit file: data = file.read() do something with data
Yous tin can too call upon other methods while using this statement. For instance, you lot can do something like loop over a file object:
westith open up("verse form.txt") every bit f: for line in f: print line,
You lot'll likewise notice that in the in a higher place case we didn't use the " file.close() " method because the with statement will automatically call that for u.s. upon execution. It really makes things a lot easier, doesn't it?
Splitting Lines in a Text File
As a final example, let's explore a unique part that allows you to split the lines taken from a text file. What this is designed to do, is dissever the string independent in variable data whenever the interpreter encounters a space character.
Just but considering nosotros are going to employ it to split lines after a space graphic symbol, doesn't mean that'southward the only way. You can really split your text using any character you wish – such as a colon, for instance.
The lawmaking to do this (also using a with statement) is:
with open( " hello.text ", "r") as f: data = f.readlines () for line in data: words = line.split () impress words
If yous wanted to use a colon instead of a infinite to split your text, you would just change line.split() to line.dissever(":").
The output for this will exist:
["hello", "world", "how", "are", "y'all", "today?"] ["today", "is", "Saturday"]
The reason the words are presented in this mode is because they are stored – and returned – equally an assortment. Exist sure to remember this when working with the split role.
Decision
Reading and writing files in Python involves an agreement of the open up() method. By taking advantage of this method's versatility, it's possible to read, write, and create files in Python.
Files Python can either exist text files or binary files. Information technology'southward too possible to open and edit prototype information using the Pillow module.
One time a file's data is loaded in Python, in that location's virtually no finish to what can be done with it. Programmers oftentimes work with a large number of files, using programs to generate them automatically.
Every bit with whatsoever lesson, in that location is but so much that can exist covered in the space provided. Hopefully you lot've learned plenty to go started reading and writing files in Python.
More Reading
Official Python Documentation – Reading and Writing Files
Python File Treatment Cheat Sheet
Recommended Python Training
Course: Python 3 For Beginners
Over 15 hours of video content with guided pedagogy for beginners. Learn how to create real earth applications and master the basics.
Source: https://www.pythonforbeginners.com/files/reading-and-writing-files-in-python
0 Response to "Python Read Numbers Text File Add Sum"
Post a Comment